기능개발
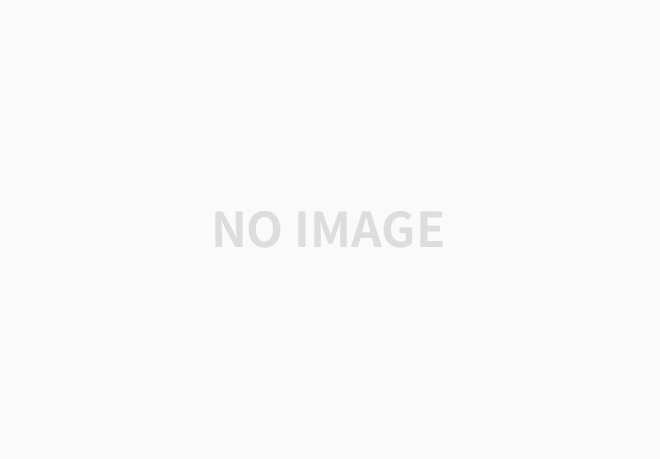
조건에 맞는 값들을 queue에 쌓아두고
while문을 이용하여 모두 제거하는 식의 문제이다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
function solution(progresses, speeds) {
var answer = [];
let counter = 0;
while(progresses.length) {
for(let i = 0; i<progresses.length; i++){
progresses[i] += + speeds[i]
}
while(progresses[0] >= 100){
progresses.shift();
speeds.shift();
counter++
}
if(counter) {
answer.push(counter);
counter=0;
}
}
return answer;
}
|
주식가격
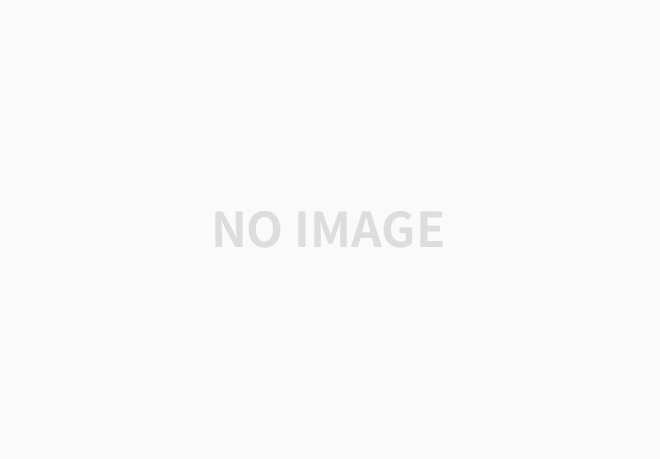
설명 없음
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
#include <string>
#include <vector>
#include <iostream>
using namespace std;
vector<int> solution(vector<int> prices) {
vector<int> answer;
for(int i =0; i<prices.size(); i++) {
int count = 0;
for(int j=i; j<prices.size(); j++) {
if(prices[i] > prices[j]){
answer.push_back(count);
break;
}
if(j == prices.size()-1) {
answer.push_back(count);
break;
}
count++;
}
}
return answer;
}
|
계산기
문자열로 입력된 수식을 연산하고 결과값을 출력하는 코드를 작성하시오. (eval 사용 금지)
input: "1+7*(15-1)/2"
output: 50
스택의 난이도 있는 문제이다.
스택의 어려움은 조건이 많기 때문인데 사칙연산은 순서에 있어서 많은 조건이 있기 때문이다.
계산 방법은
현재의 중위 표기법으로 된 식을 후위 표기법으로 바꾼 후 계산한다.
그렇기 때문에 크게 두가지 코드로 나누었다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
|
const question = "1+7*(15-1)/2"
const stack = []
const output = []
function postfix(question) {
const arr = []
let tempNum = ""
for(let index in question) {
let nowNumCheck = question[index].match(/[0-9]/)
if(arr.length) {
let lastNumCheck = arr[arr.length-1].match(/[0-9]/)
if(lastNumCheck && !nowNumCheck) {
arr.push(question[index])
}else if(!lastNumCheck && nowNumCheck){
arr.push(question[index])
}else if(!lastNumCheck && !nowNumCheck) {
arr.push(question[index])
}else{
tempNum = arr.pop() + question[index]
arr.push(tempNum)
}
}else {
arr.push(question[index])
}
}
for(value of arr) {
if(!value.match(/[0-9]/)) {
if(!stack.length) {
stack.push(value)
}else{
if(value == "(") {
stack.push(value);
}
if(value == ")") {
while(stack.length) {
let target = stack.pop()
if(target != "(") {
output.push(target)
}else{
break;
}
}
if(output[output.length-1] == "+" || output[output.length-1] == "-") {
while(true){
if(stack[stack.length-1] == "*" || stack[stack.length-1] == "/") {
output.push(stack.pop());
}else{
break;
}
}
}
}
if(stack.indexOf("(") >= 0) {
if(value != "(")
stack.push(value);
}else{
if(value == "+" || value == "-") {
if(
stack[0] == "*" ||
stack[0] == "/" ||
stack[0] == "+" ||
stack[0] == "–" ) {
for(let i =0; i<stack.length; i++) {
output.push(stack.pop())
}
stack.push(value);
}
}
if(value == "*" || value == "/") {
if(stack[0] == "*" || stack[0] == "/") {
output.push(stack.pop())
stack.push(value);
}else if(stack[0] == "+" || stack[0] == "-") {
stack.push(value);
}
}
}
}
}else{
output.push(value)
}
}
while(stack.length) {
output.push(stack.pop());
}
}
postfix(question)
|
위 코드는 후위표기식을 작성하는 코드이다.
후위 표기식 조건은
1. 현재 타겟이 ( 일 경우에는
1-1. 타겟이 ) 이전까지의 모든 연산자를 스택에 입력한다.
1-2 타겟이 ) 일 경우 괄호안의 모든 연산자를 pop 하여 후위표기식에 입력한다.
2. 현재타겟이 (가 아닐 경우는
2-1. + 혹은 - 가 타겟 일떄
2-1-1. 만약 이전 값이 + - * / 일 경우 스택에 담겨진 모든 연산자를 pop하여 후위표기식에 넣는다.
2-2 * 혹은 -가 타겟일 때
2-2-1. 스택의 top이 * 혹은 / 라면 스택의 모든 * 와 / 를 pop하여 후위표기식에 넣는다.
2-2-2 스택의 top이 + 혹은 - 라면 스택에 해당 타겟을 push한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
function calculator(output){
const outputArr = output
for(value of outputArr) {
if(value.match(/[0-9]/)) {
calStack.push(value)
}else{
let a = Number(calStack.pop())
let b = Number(calStack.pop())
if(value=="+") calStack.push(b + a)
if(value == "-") calStack.push(b - a)
if(value == "*") calStack.push(b * a)
if(value == "/") Math.round(calStack.push(b / a))
}
}
return calStack[0]
}
|
위 코드는 후위표기식을 받아 계산한다.
타겟이 숫자일 경우에는 stack에 담고
타겟이 연산자일 경우 stack에서 숫자를 pop 하여 연산해 push 한다.
'Algorithm' 카테고리의 다른 글
탐욕법 (0) | 2019.08.06 |
---|---|
[DFS/BFS] 여행경로 (0) | 2019.07.28 |
[스택/큐] 프린터, 탑, 쇠막대기, 다리를 지나는 트럭 (0) | 2019.07.26 |
[DP] 가장 큰 정사각형 찾기/ 땅따먹기 (0) | 2019.07.19 |
[완전탐색] 소수 찾기 (0) | 2019.07.17 |